var multiparty = require('multiparty')
var http = require('http')
let fs = require('fs')
/*
Creates a new form. Options:
encoding - sets encoding for the incoming form fields. Defaults to utf8.
maxFieldsSize - Limits the amount of memory all fields (not files) can allocate in bytes. If this value is exceeded, an error event is emitted. The default size is 2MB.
maxFields - Limits the number of fields that will be parsed before emitting an error event. A file counts as a field in this case. Defaults to 1000.
maxFilesSize - Only relevant when autoFiles is true. Limits the total bytes accepted for all files combined. If this value is exceeded, an error event is emitted. The default is Infinity.
autoFields - Enables field events and disables part events for fields. This is automatically set to true if you add a field listener.
autoFiles - Enables file events and disables part events for files. This is automatically set to true if you add a file listener.
uploadDir - Only relevant when autoFiles is true. The directory for placing file uploads in. You can move them later using fs.rename(). Defaults to os.tmpdir().
这个服务端有二个需要注意的地方
1.var form = new multiparty.Form();
这里的文件路径并不会自动创建,需要用户自己在项目的根目录中创建该路径,否则就会报文件路径不存在的错误。
form.uploadDir = './file' // !important
2.var file = files.upload[0]
这行代码中,upload是form表单中的input组件的name值,用来获取文件
*/
http.createServer(function (req, res) {
if (req.url === '/upload' && req.method === 'POST') {
// parse a file upload
var form = new multiparty.Form()
form.uploadDir = './file' // !important
console.log('开始上传')
form.parse(req, function (err, fields, files) {
if (err) {
console.log('上传出错')
return false
} else {
console.log('上传完毕')
var file = files.upload[0]
console.log(file)
console.log(file.path)
fs.rename(file.path, './file/' + file.originalFilename, (err) => {
if (err) {
console.log('修改失败')
res.writeHead(200, {'content-type': 'text/plain;charset=utf-8'})
res.end("{'status':200, 'message': '上传失败!'}")
} else {
console.log('修改名字成功')
res.writeHead(200, {'content-type': 'text/plain;charset=utf-8'})
res.end("{'status':400, 'message': '上传成功!'}")
}
})
}
})
}
// show a file upload form
res.writeHead(200, {'content-type': 'text/html; charset=UTF-8'})
res.end(
'<form action="/upload" enctype="multipart/form-data" method="post">' +
'<input type="text" name="title"><br>' +
'<input type="file" name="upload" multiple="multiple"><br>' +
'<input type="submit" value="Upload">' +
'</form>'
)
}).listen(5555)
console.log('http://localhost:5555')
项目结构
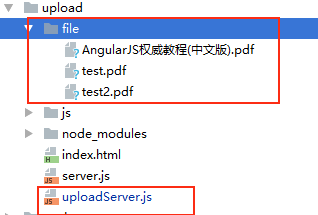